Max retries exceeded with URL in requests in Python
If you are facing this error then the issue is not on server side and it is coming from your code.
URL parameters in Python can be simple to use, but sometimes a problem arises. If the retries are exceeded with URL in requests, this article will walk you through the steps to fix this issue.
If you have ever come across the following error when using the requests library in Python: "Max retries exceeded with URL", then this article is for you.
In this article, we will take a look at what this error means and how you can fix it.
The "Max retries exceeded with URL" error is caused by the fact that therequests library is unable to establish a connection to the server. This can be due to a number of reasons, such as:
- The server is down or unreachable.
- There is a problem with the network connection.
- The server is taking too long to respond.
The first thing you should do when seeing this error is check whether the server is up and running. You can do this by trying to access the website in your browser or using a tool like Pingdom.
If the server appears to be up and running, then the next step is to check your network connection. This can be done by restarting your router or modem. If that does not work, then you may need to contact your ISP for help.
Once you have ruled out any networking issues, the next step is to check if the server is taking too long to respond. This can be caused by a number of factors, such as:
- The website is experiencing high traffic levels.
- The website is under construction or undergoing maintenance.
- There is a problem with the website
The Problem
If you're seeing the error "Max retries exceeded with URL" in your Python requests, it means that your program is trying to request a URL that is taking too long to respond. This can be caused by a number of factors, including:
-The server you're trying to reach is down or slow -There's a problem with your network connection -The URL you're trying to reach is invalid
If you see this error, the first thing you should do is check the status of the server you're trying to reach. If it's up and running, try again later. If the server is down, there's not much you can do except wait for it to come back online.
Next, check your network connection. Make sure you're connected to the internet and that your firewall isn't blocking requests from Python. If everything looks good on your end, try reaching out to the owner of the website or service you're trying to access and let them know there's a problem.
Finally, if none of these solutions work, it's possible that the URL you're trying to reach is simply invalid. Double-check the spelling and try again. If all else fails, contact customer support for help troubleshooting the issue.
Solutions to fix Max retries exceeded with URL in requests
There are a few potential solutions for this issue. One is to increase the number of retries allowed for the request. Another is to change the URL used for the request. Finally, you could also look into using a different Python library for making requests.
The Python requests library provides a retry method to handle this situation. It will keep on trying to make the request until it gets an HTTP 200 response code.
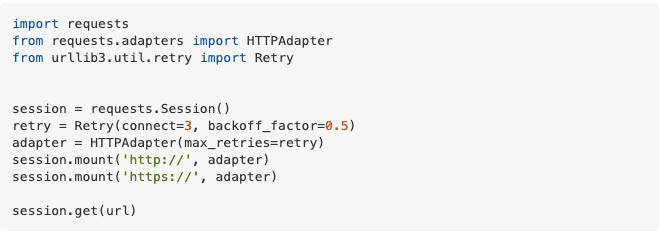
This is done by specifying how many times you want to retry and for how long you want to wait in between each retry attempt.
I hope this article has helped you understand how to fix the "Max retries exceeded with URL" error in Python. This is a common error that can be fixed by simply increasing the number of retries in your code. By doing this, you will be able to successfully connect to the URL and retrieve the data you need.